Practical Guide to Centering a Div: Essential Techniques for 2025
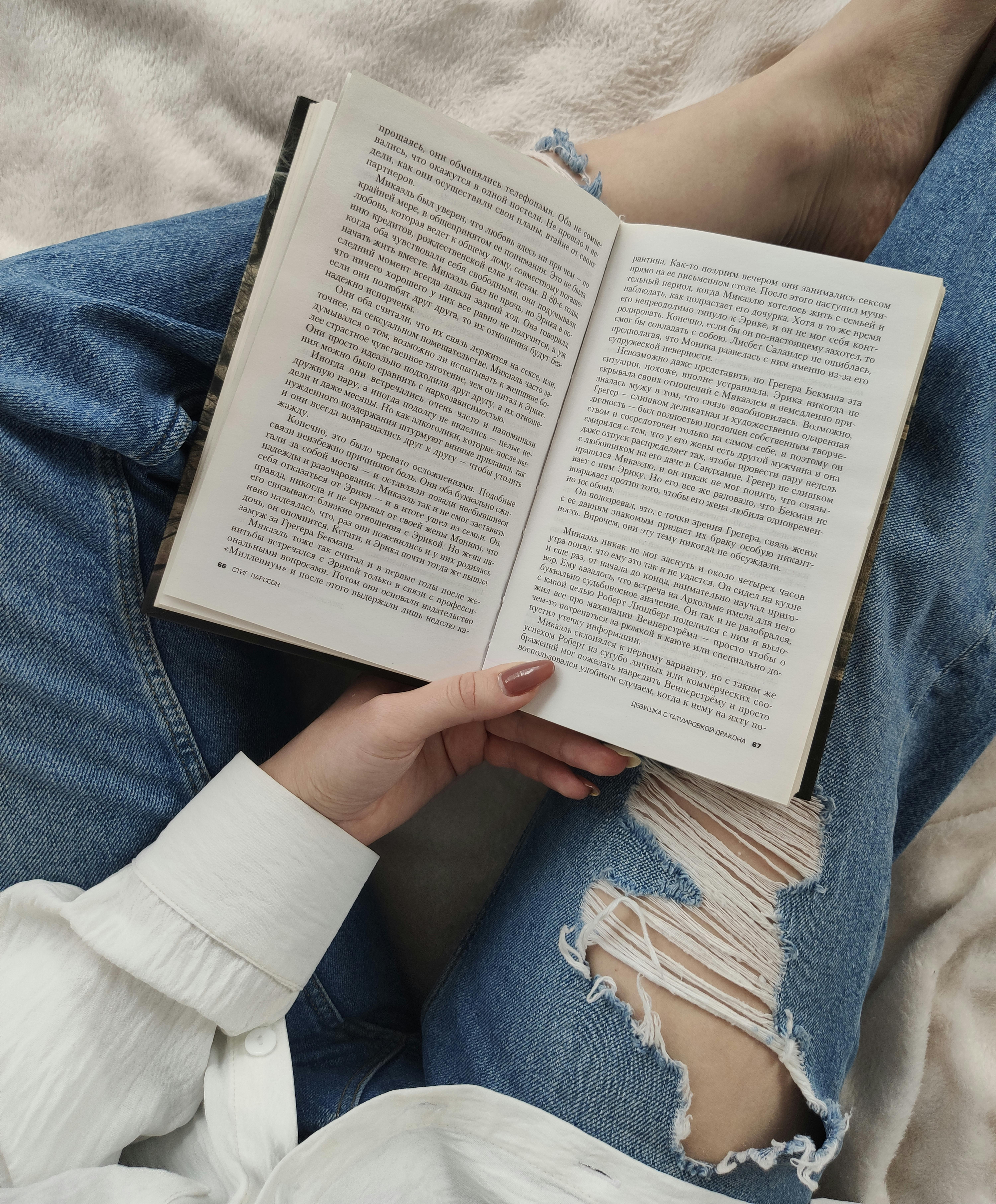
Effective Ways to Center a Div: Proven Techniques for 2025
In modern web design, achieving the perfect alignment of elements is crucial for both aesthetic appeal and user experience. Centering a div is a fundamental task that many developers encounter, and with various techniques available, knowing which methods to utilize can significantly enhance the design quality of your webpage. In this article, we will explore a range of effective ways to center a div using CSS, HTML layout strategies, and responsive design practices. By mastering these techniques, you can create visually appealing and accessible content that engages users effectively.
We will cover the following techniques:
- Using Flexbox and Grid for centering divs
- Implementing margin auto for horizontal alignment
- Vertical centering techniques
- Responsive design strategies for different screen sizes
- Best practices for cross-browser compatibility
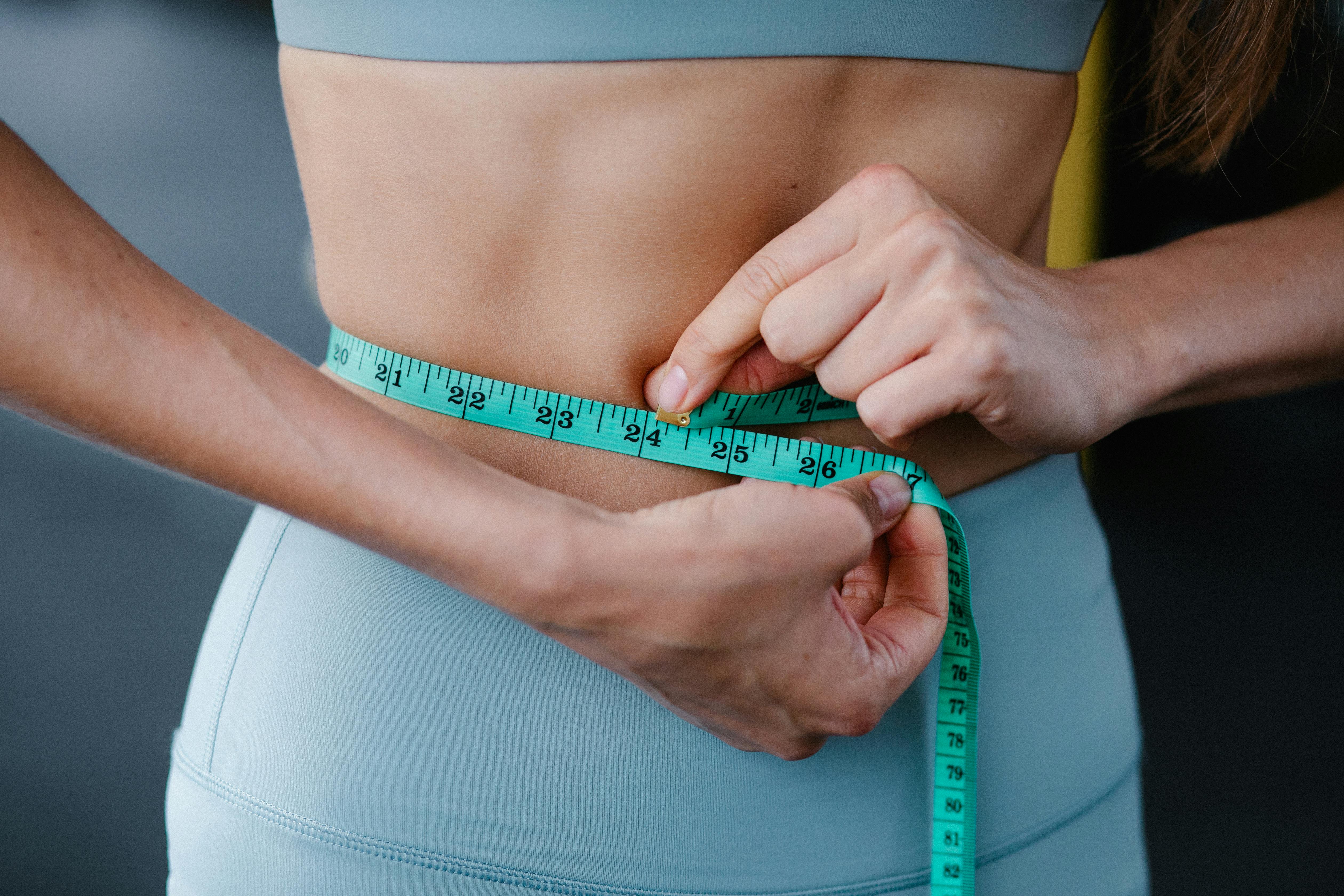
Mastering Flexbox Centering Techniques
Understanding Flexbox Layout
Flexbox, or the Flexible Box Layout, is a powerful CSS layout mode that makes it easy to design flexible and responsive layouts. By setting the display property of a container to display: flex;
, you can align and distribute space among items within the container.
To center a div horizontally and vertically using Flexbox, you can use the following approach:
.parent { display: flex; justify-content: center; /* Centers horizontally */ align-items: center; /* Centers vertically */ } .child { width: 50%; /* Adjust width as needed */ }
This easily centers the child div within the parent container, ensuring a flexible and adaptive layout.
Centering with Flexbox: Practical Examples
Consider the following scenario where you want to center a navigation bar within a header. By applying Flexbox techniques, you can achieve a coherent and easily navigable design.
.header { display: flex; justify-content: center; align-items: center; } .nav { display: flex; justify-content: space-around; /* Equally spaces items */ }
This example showcases how to maintain alignment while providing user-friendly navigation. Flexbox's properties simplify alignment and ensure smooth user interactions.
Common Mistakes in Flexbox Centering
While Flexbox offers powerful tools for alignment, developers can still make common errors:
- Neglecting to set appropriate widths for child elements, which can lead to unexpected results.
- Forgetting the
flex-wrap
property, which may complicate layouts in responsive designs.
By being aware of these pitfalls, you can enhance your use of Flexbox for effective layout designs.
Utilizing Grid Layout for Centering
Introduction to CSS Grid
CSS Grid Layout is another robust technique that enables web developers to create complex layouts. With a grid-based system, centering elements is as straightforward as defining grid areas and utilizing grid properties.
.parent { display: grid; place-items: center; /* Centers both horizontally and vertically */ }
This method allows for straightforward centering and fine control over the layout with minimal code.
Creating Responsive Grid Layouts
Grids are inherently responsive; using media queries can enhance their functionality further. For instance, you can adjust grid rows and columns based on the viewport size to maintain a balanced look.
@media (max-width: 600px) { .parent { grid-template-columns: 1fr; /* Stacks items on smaller screens */ } }
This technique ensures that your design remains visually appealing across devices, providing a seamless user experience.
Best Practices for Using Grid
Implementing best practices in CSS Grid layout can optimize your designs:
- Always define a grid gap for spacing.
- Utilize responsive units and media queries to maintain design integrity.
These practices will result in user-friendly designs that adapt gracefully across various screen sizes.
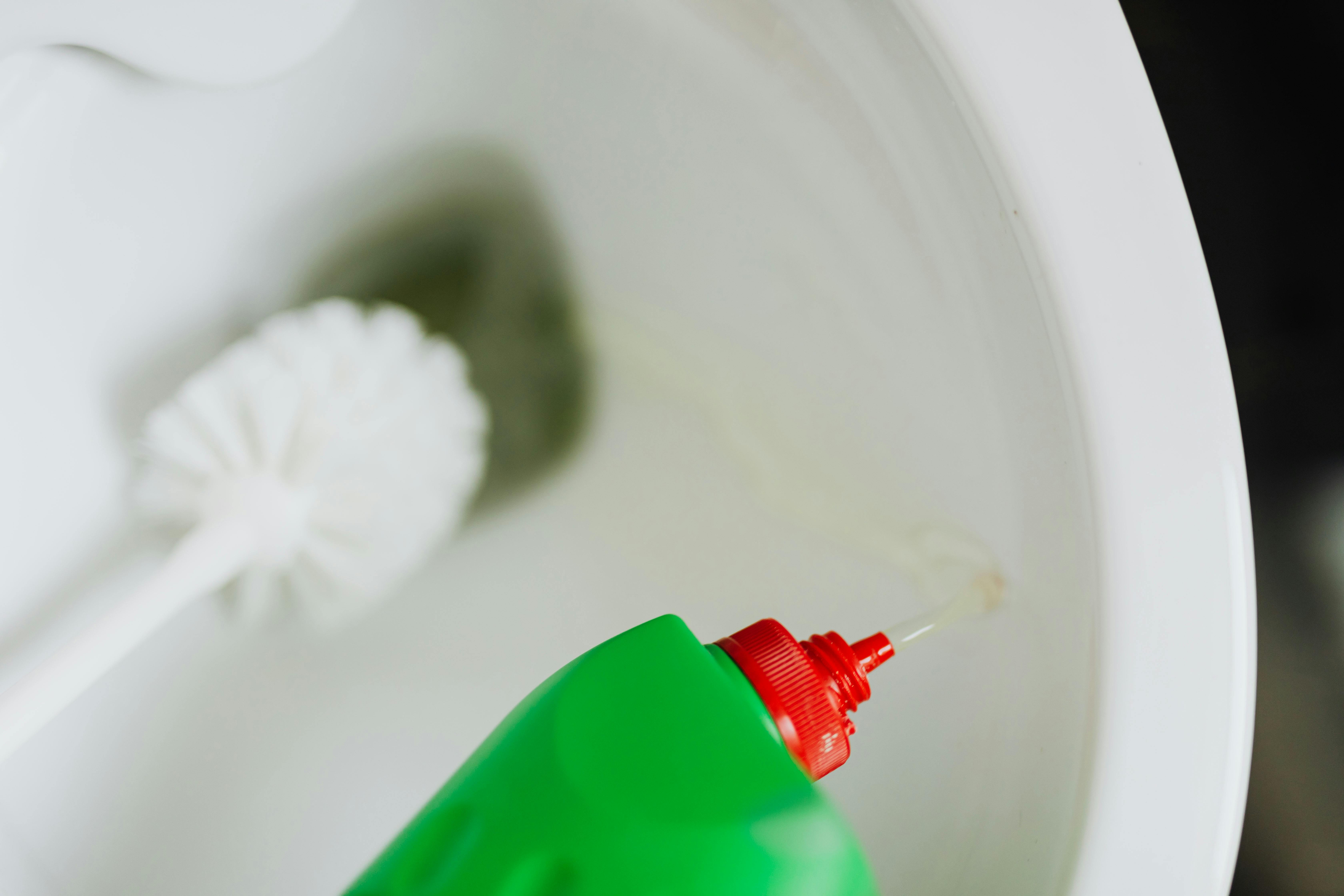
Horizontal Centering Techniques
Implementing Margin Auto for Centering
One of the simplest ways to center a div horizontally is by using the margin: auto;
CSS property. This method works well for block elements with a defined width.
.child { width: 50%; margin: 0 auto; /* Centers horizontally */ }
This approach ensures that the element takes only the specified width and automatically allocates equal margins on both sides, centering it perfectly within its parent.
Inline-Block Centering
For inline or inline-block elements, centering can be achieved using text-align: center;
on the parent and setting the child elements as inline-block:
.parent { text-align: center; } .child { display: inline-block; /* Centered within parent */ }
This technique is effective for vertically aligning multiple inline elements while keeping them centered.
Common Pitfalls in Horizontal Centering
While using margin auto is straightforward, it's easy to overlook certain details, such as:
- Not setting a width for block elements, leading to unexpected alignment.
- Incorrectly using floats, which can disrupt the normal flow of elements.
Be mindful of these mistakes to ensure effective horizontal centering in your designs.
Vertical Centering Techniques
Using CSS Flexbox for Vertical Centering
Vertical alignment is sometimes trickier than horizontal, but Flexbox simplifies this process dramatically. By setting align-items: center;
, you can vertically center any child within a flex container.
It's an especially effective technique for aligning elements across various devices and resolutions, ensuring consistent user experiences.
Absolute Positioning for Centering
Another approach to vertically center elements involves using absolute positioning. By setting the element's position to absolute and defining top and left properties:
.child { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); /* Perfectly centers the element */ }
This method allows for precise control over placement but may require careful handling in responsive designs.
Responsive Vertical Centering
Responsive design is crucial for creating accessible web applications. CSS Grid and Flexbox techniques should include media queries to adjust the vertical alignment based on screen sizes, maintaining a balance and visually appealing layout.
Ensuring Cross-Browser Compatibility
Best Practices for Cross-Browser Styling
When developing web applications, ensuring that centering techniques work across all major browsers is essential for creating user-friendly interfaces. Always test layouts on various browsers, adjusting CSS properties accordingly to maintain functionality.
Common Compatibility Issues
While most modern browsers support the latest CSS features, older versions may not. Be aware of:
- Flexbox support in older browsers, which may require fallbacks or alternative methods.
- Grid compatibility, ensuring smooth functionality on all platforms.
Addressing these issues early on will help maintain user satisfaction across all devices.
Q&A: Centering a Div in Web Development
What is the best way to center a div?
The best method depends on the context. Flexbox offers flexible solutions for responsive designs, while margin auto is effective for fixed-width block elements. Choose based on your layout needs.
Can I center a div with CSS Grid?
Yes, CSS Grid allows for straightforward centering using the place-items: center;
property, making it a powerful tool for responsive layouts.
Is vertical centering more challenging than horizontal centering?
It can be, as vertical centering often requires additional considerations, like using Flexbox or absolute positioning. Understanding both styles greatly enhances your layout capabilities.
How can I ensure my designs are mobile-friendly?
Utilizing responsive design techniques and media queries ensures that your layouts adapt to various screen sizes, maintaining functionality and aesthetics.
Are there any tools to assist in centering elements?
Yes, many CSS frameworks and web development tools provide built-in classes and utilities for centering elements, simplifying the process for developers.